2025.05
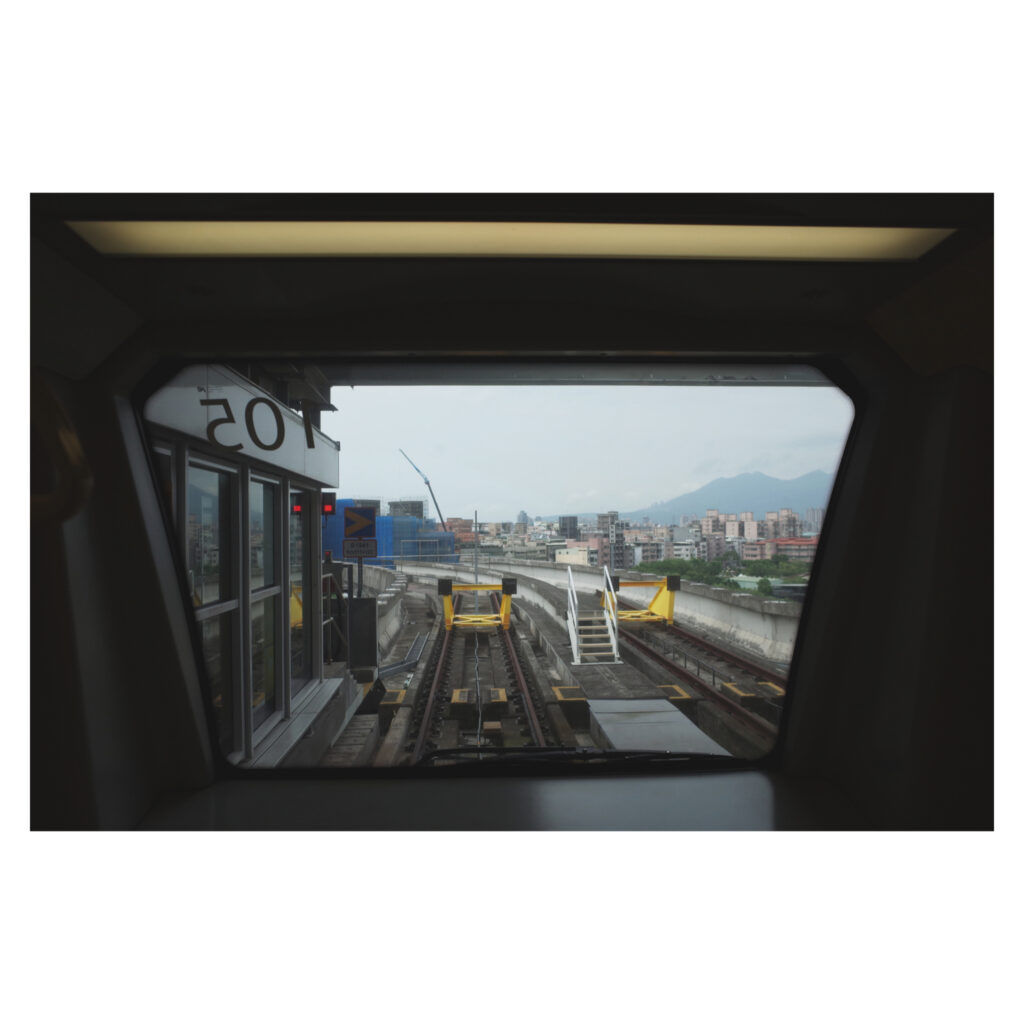
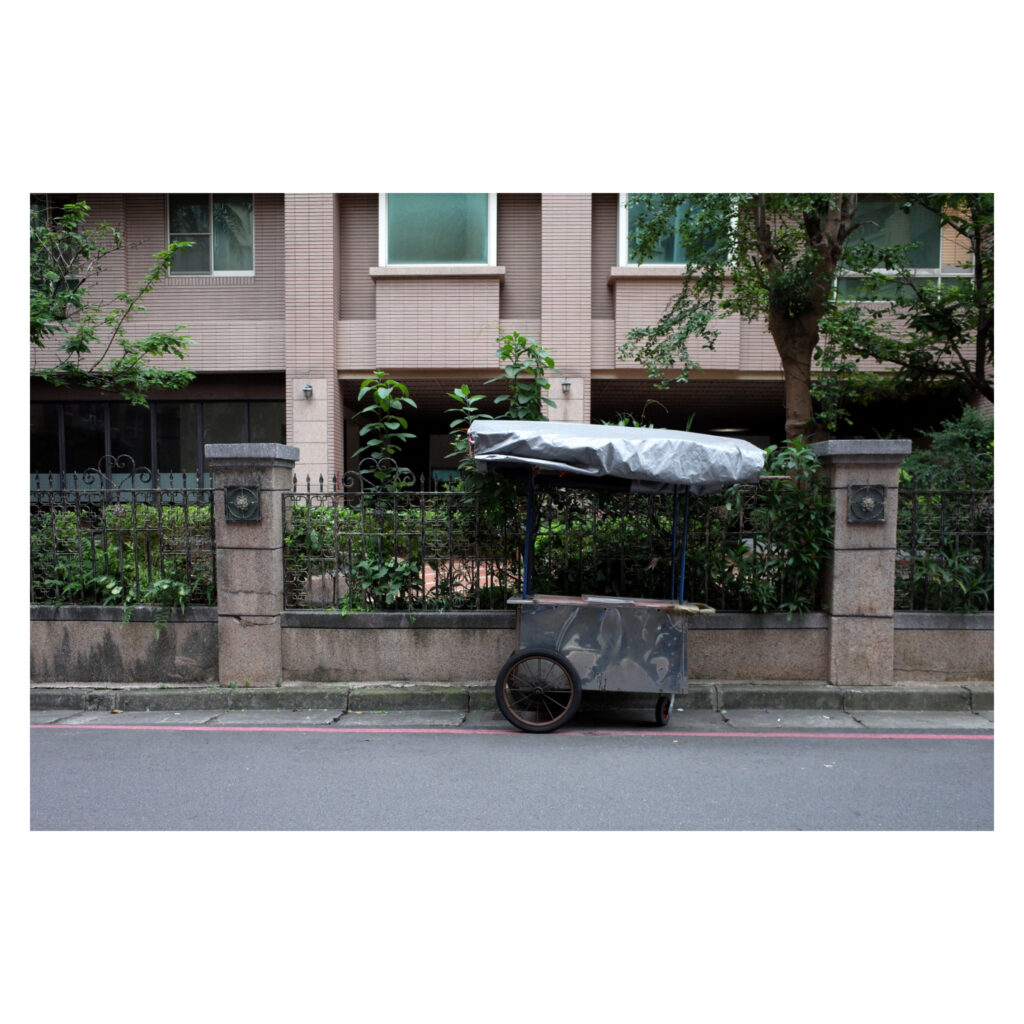
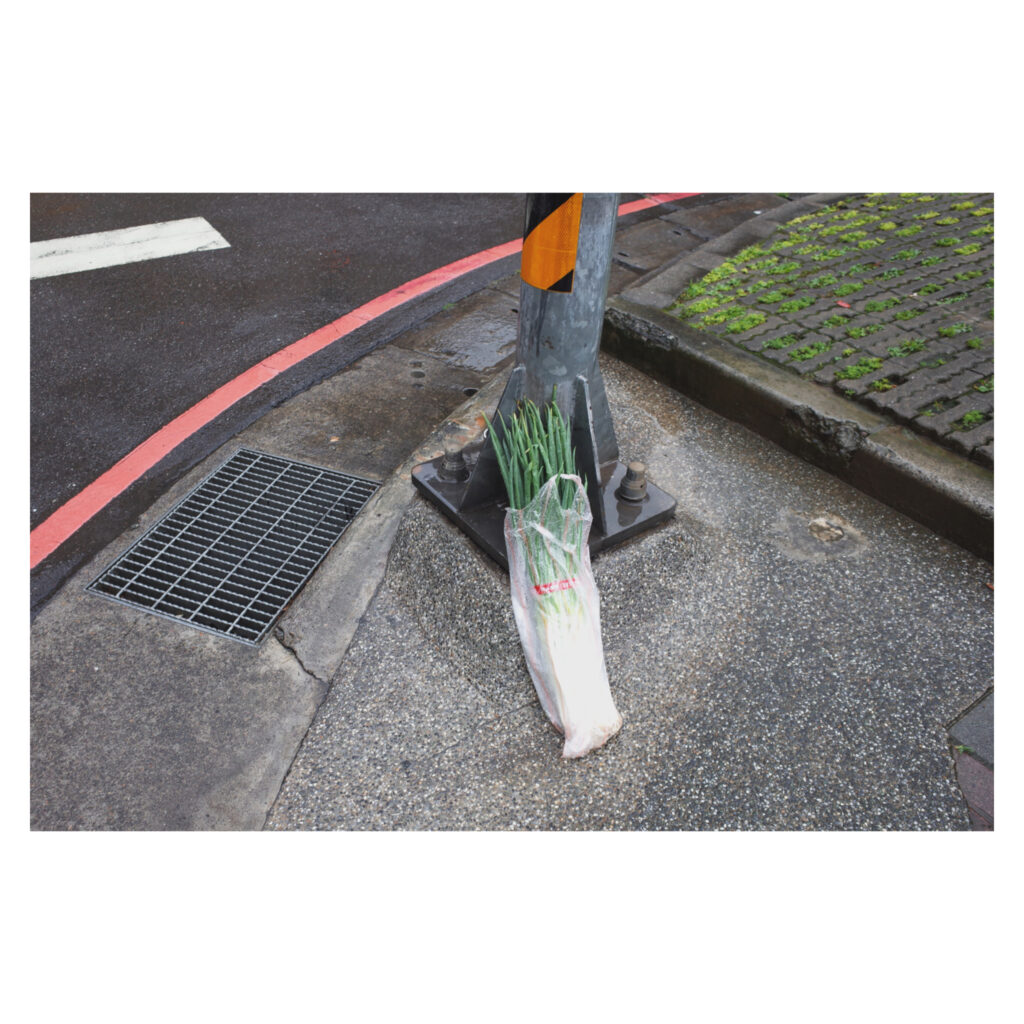
安裝 acme.sh 取得 SSL 憑證。
我用的方式是 Standalone tls alpn mode,port 443 必須先空出來,不能被使用。
# 一開始要拿憑證的時候一直出現錯誤
# 後來在網路上看到可以加 --set-default-ca --server letsencrypt
# 加上後就成功了
acme.sh --set-default-ca --server letsencrypt --issue --alpn -d YOUR.DOMAIN.COM
Postfix 設定方式如下:
smtpd_use_tls = yes
smtpd_tls_auth_only = no
smtpd_tls_cert_file = /etc/letsencrypt/..../fullchain.pem
smtpd_tls_key_file = /etc/letsencrypt/..../privkey.pem
tls_random_source = dev:/dev/urandom
smtpd_tls_received_header = yes
smtp_use_tls = yes
smtp_tls_note_starttls_offer = yes
再修改 /etc/postfix/master.cf,下面三行拿掉註解。
smtps inet n - n - - smtpd
-o smtpd_tls_wrappermode=yes
-o smtpd_sasl_auth_enable=yes
重新啟動 Postfix
systemctl restart postfix
記得要修改 Firewall 設定,讓 TCP port 465 可以通行(SMTP uses port 25, but SSL/TLS encrypted SMTP uses port 465)。
再來要修改 SELinux 規則,因為 acme.sh 的相關檔案都放在 root 家目錄,其他服務要讀取會有問題。
# 安裝工具
yum install setroubleshoot*
再到 /var/log/messages 看相關訊息,跟著訊息下指令安裝 SELinux 規則,規則安裝好後,似乎要重新開機才可以正常運作。
參考連結:
我的 Node.js app 是用 PM2 啟動,MySQL 是放在 Docker。
因為 PM2 啟動 app 比 Docker 快,所以會出現錯誤訊息(資料庫連線錯誤)。
請 ChatGPT 幫忙,延後 PM2 啟動執行 app,方法如下:
先建一個 timer 檔案: /etc/systemd/system/myservice-delay.timer
內容:
[Unit]
Description=Delay start for myservice
[Timer]
OnBootSec=60s
Unit=myservice.service
[Install]
WantedBy=timers.target
這個 Timer 的意思是:開機後 60 秒,才觸發 myservice.service
啟用 Timer:
sudo systemctl daemon-reload
sudo systemctl enable myservice-delay.timer
但有一個地方要注意,原本用 PM2 產生的 service 要停止開機啟動。
sudo systemctl disable myservice
location / {
# First attempt to serve request as file, then
# as directory, then fall back to displaying a 404.
#try_files $uri $uri/ =404;
allow 192.168.0.0/24;
deny all;
proxy_pass http://127.0.0.1:3000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}